rsa加密解密c语言算法_C和C ++中的RSA算法(加密和解密)
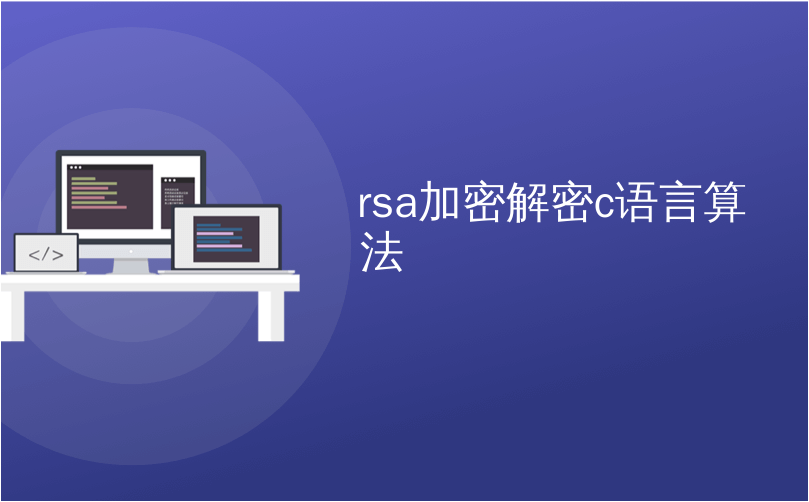
rsa加密解密c语言算法
Here you will learn about RSA algorithm in C and C++.
在这里,您将了解C和C ++中的RSA算法。
RSA Algorithm is used to encrypt and decrypt data in modern computer systems and other electronic devices. RSA algorithm is an asymmetric cryptographic algorithm as it creates 2 different keys for the purpose of encryption and decryption. It is public key cryptography as one of the keys involved is made public. RSA stands for Ron Rivest, Adi Shamir and Leonard Adleman who first publicly described it in 1978.
RSA算法用于在现代计算机系统和其他电子设备中加密和解密数据。 RSA算法是一种非对称密码算法,因为它创建2个不同的密钥用于加密和解密。 这是公钥密码术,因为其中涉及的密钥之一是公开的。 RSA代表Ron Rivest,Adi Shamir和Leonard Adleman,他们于1978年首次公开描述它。
RSA makes use of prime numbers (arbitrary large numbers) to function. The public key is made available publicly (means to everyone) and only the person having the private key with them can decrypt the original message.
RSA利用质数(任意大数)起作用。 公钥是公开可用的(对所有人均适用),只有拥有私钥的人才能解密原始消息。
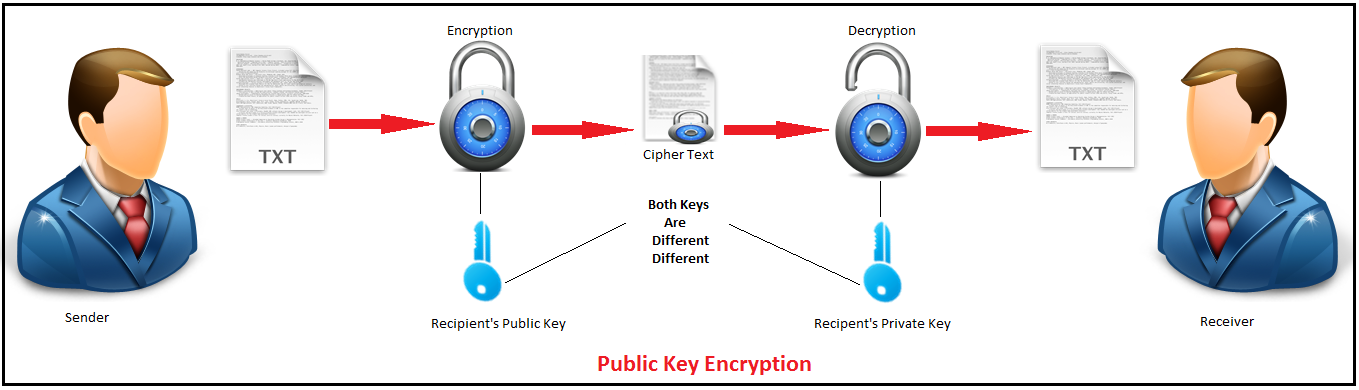
图片来源
RSA算法的工作 (Working of RSA Algorithm)
RSA involves use of public and private key for its operation. The keys are generated using the following steps:-
RSA涉及使用公钥和私钥进行操作。 密钥使用以下步骤生成:
Two prime numbers are selected as p and q
选择两个质数作为p和q
n = pq which is the modulus of both the keys.
n = pq ,这是两个键的模数。
Calculate totient = (p-1)(q-1)
计算总含量=(p-1)(q-1)
Choose e such that e > 1 and coprime to totient which means gcd (e, totient) must be equal to 1, e is the public key
选择e ,使e> 1并乘以totoient ,这意味着gcd(e,totient)必须等于1 , e是公钥
Choose d such that it satisfies the equation de = 1 + k (totient), d is the private key not known to everyone.
选择d使其满足等式de = 1 + k(totient) , d是每个人都不知道的私钥。
Cipher text is calculated using the equation c = m^e mod n where m is the message.
使用等式c = m ^ e mod n计算密文,其中m是消息。
With the help of c and d we decrypt message using equation m = c^d mod n where d is the private key.
借助c和d,我们使用等式m = c ^ d mod n解密消息,其中d是私钥。
Note: If we take the two prime numbers very large it enhances security but requires implementation of Exponentiation by squaring algorithm and square and multiply algorithm for effective encryption and decryption. For simplicity the program is designed with relatively small prime numbers.
注意:如果我们将两个素数取得非常大,则会提高安全性,但需要通过平方算法和平方乘算法来实现幂运算,以实现有效的加密和解密。 为简单起见,程序设计为具有相对较小的质数。
Below is the implementation of this algorithm in C and C++.
下面是该算法在C和C ++中的实现。
C语言RSA算法程序 (Program for RSA Algorithm in C)
//Program for RSA asymmetric cryptographic algorithm
//for demonstration values are relatively small compared to practical application
#include<stdio.h>
#include<math.h>
//to find gcd
int gcd(int a, int h)
{
int temp;
while(1)
{
temp = a%h;
if(temp==0)
return h;
a = h;
h = temp;
}
}
int main()
{
//2 random prime numbers
double p = 3;
double q = 7;
double n=p*q;
double count;
double totient = (p-1)*(q-1);
//public key
//e stands for encrypt
double e=2;
//for checking co-prime which satisfies e>1
while(e<totient){
count = gcd(e,totient);
if(count==1)
break;
else
e++;
}
//private key
//d stands for decrypt
double d;
//k can be any arbitrary value
double k = 2;
//choosing d such that it satisfies d*e = 1 + k * totient
d = (1 + (k*totient))/e;
double msg = 12;
double c = pow(msg,e);
double m = pow(c,d);
c=fmod(c,n);
m=fmod(m,n);
printf("Message data = %lf",msg);
printf("\np = %lf",p);
printf("\nq = %lf",q);
printf("\nn = pq = %lf",n);
printf("\ntotient = %lf",totient);
printf("\ne = %lf",e);
printf("\nd = %lf",d);
printf("\nEncrypted data = %lf",c);
printf("\nOriginal Message Sent = %lf",m);
return 0;
}
C ++中的RSA算法程序 (Program for RSA Algorithm in C++)
//Program for RSA asymmetric cryptographic algorithm
//for demonstration values are relatively small compared to practical application
#include<iostream>
#include<math.h>
using namespace std;
//to find gcd
int gcd(int a, int h)
{
int temp;
while(1)
{
temp = a%h;
if(temp==0)
return h;
a = h;
h = temp;
}
}
int main()
{
//2 random prime numbers
double p = 3;
double q = 7;
double n=p*q;
double count;
double totient = (p-1)*(q-1);
//public key
//e stands for encrypt
double e=2;
//for checking co-prime which satisfies e>1
while(e<totient){
count = gcd(e,totient);
if(count==1)
break;
else
e++;
}
//private key
//d stands for decrypt
double d;
//k can be any arbitrary value
double k = 2;
//choosing d such that it satisfies d*e = 1 + k * totient
d = (1 + (k*totient))/e;
double msg = 12;
double c = pow(msg,e);
double m = pow(c,d);
c=fmod(c,n);
m=fmod(m,n);
cout<<"Message data = "<<msg;
cout<<"\n"<<"p = "<<p;
cout<<"\n"<<"q = "<<q;
cout<<"\n"<<"n = pq = "<<n;
cout<<"\n"<<"totient = "<<totient;
cout<<"\n"<<"e = "<<e;
cout<<"\n"<<"d = "<<d;
cout<<"\n"<<"Encrypted data = "<<c;
cout<<"\n"<<"Original Message sent = "<<m;
return 0;
}
Output
输出量
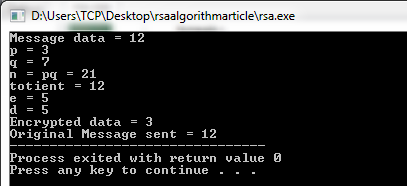
This article is submitted by Rahul Maheshwari. You can connect with him on facebook.
本文由Rahul Maheshwari提交。 您可以在 Facebook上与他建立联系。
Comment below if you have any queries related to above program for rsa algorithm in C and C++.
如果您对C和C ++中的rsa算法的上述程序有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/03/rsa-algorithm.html
rsa加密解密c语言算法